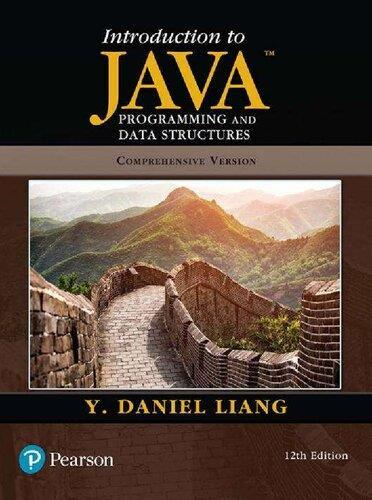
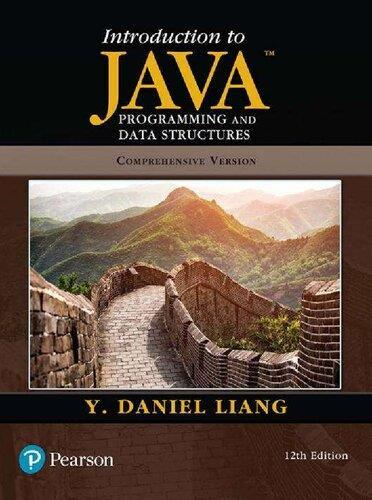
"Introduction to Java Programming and Data
Structures, Comprehensive Version" (12th Edition) by Y. Daniel Liang is an extensive textbook designed to teach programming in Java and data structures using a comprehensive approach. Here’s a detailed summary of the book’s key concepts and topics:
1. Introduction to Java Programming
1.1 Basics of Java
• Java Overview: Introduction to the Java programming language, its history, and its role as a widely used, high-level, object-oriented language.
• Java Development Kit (JDK): Components of the JDK, including the compiler (javac), Java Runtime Environment (JRE), and the Java Virtual Machine (JVM).
• First Java Program: Step-by-step instructions on writing, compiling, and running a basic Java program. Emphasis on the structure of a Java program, including classes, methods, and the main method.
1.2 Variables and Data Types
• Primitive Data Types: Discussion on Java’s primitive data types, including int, double, char, and boolean.
• Variables: How to declare and initialize variables, variable scope, and the concept of type conversion and casting.
1.3 Operators and Expressions
• Operators: Arithmetic, relational, logical, and bitwise operators. Understanding operator precedence and associativity.
• Expressions: Formation and evaluation of expressions, including the use of parentheses to control evaluation order.
1.4
Control Structures
• Conditional Statements: Use of if, else if, and else statements to perform conditional execution.
• Loops: Implementation of loops (for, while, dowhile) to repeat code execution. Discussion on
loop control statements like break and continue.
1.5 Methods
• Defining and Calling Methods: How to define methods, pass parameters, and return values. Overloading methods and understanding method scope.
• Recursive Methods: Introduction to recursion, with examples of recursive algorithms and their applications.
2. Object-Oriented Programming
2.1 Classes and Objects
• Class Definition: Structure of a Java class, including fields, constructors, and methods.
• Creating Objects: Instantiation of objects using the new keyword and invoking methods on objects.
• Encapsulation: Use of access modifiers (private, public, protected) to control access to class members.
2.2 Inheritance
• Superclass and Subclass: Concepts of inheritance, where a subclass inherits properties and methods from a superclass.
• Method Overriding: How to override methods in a subclass to provide specific implementations.
• The super Keyword: Usage of the super keyword to access superclass members and constructors.
2.3 Polymorphism
• Method Overloading: Ability to define multiple methods with the same name but different parameters.
• Method Overriding: Ensuring that subclasses provide specific implementations of methods defined in superclasses.
• Dynamic Method Dispatch: Mechanism by which Java determines the method to execute at runtime based on the object’s actual type.
2.4 Abstract Classes and Interfaces
• Abstract Classes: Definition and use of abstract classes that cannot be instantiated and may contain abstract methods.
• Interfaces: How to define and implement interfaces, including the use of default and static methods in interfaces.
3. Data Structures
3.1 Arrays
• One-Dimensional Arrays: Declaration, initialization, and manipulation of singledimensional arrays.
• Multi-Dimensional Arrays: Working with twodimensional and higher-dimensional arrays.
3.2 Strings
• String Class: Methods and operations provided by the String class, including string manipulation, comparison, and conversion.
• StringBuilder and StringBuffer: Use of StringBuilder and StringBuffer for mutable
strings and their performance advantages over String.
3.3 Lists and Collections
• ArrayList: Implementation and usage of ArrayList, including methods for adding, removing, and accessing elements.
• LinkedList: Understanding LinkedList, its advantages, and use cases compared to ArrayList.
• Sets: Introduction to HashSet and TreeSet, and their use cases for storing unique elements.
• Maps: Implementation and usage of HashMap and TreeMap for key-value pair storage.
3.4 Stacks and Queues
• Stack: Implementation of the stack data structure, including operations such as push, pop, and peek.
• Queue: Implementation of the queue data structure, including operations such as enqueue, dequeue, and peek.
3.5 Trees and Graphs
• Binary Trees: Basic concepts of binary trees, including traversal methods (in-order, pre-order, post-order).
• Binary Search Trees (BST): Implementation and usage of BSTs for efficient data searching and insertion.
• Graphs: Introduction to graph theory, representation of graphs, and basic graph algorithms like depth-first search (DFS) and breadth-first search (BFS).
4. Advanced Topics
4.1 Exception Handling
• Try-Catch-Finally: Syntax and usage of try, catch, and finally blocks for handling exceptions.
• Custom Exceptions: Creation and throwing of custom exceptions to handle specific error conditions.
• Exception Hierarchy: Understanding Java’s exception hierarchy, including checked and unchecked exceptions.
4.2 File I/O
• Reading and Writing Files: Use of FileReader, FileWriter, BufferedReader, and BufferedWriter for file operations.
• Serialization: Concepts of serialization and deserialization for saving and restoring objects.
4.3 Threads and Concurrency
• Thread Creation: Creating and running threads using the Thread class and Runnable interface.
• Synchronization: Mechanisms for synchronizing threads to prevent concurrent access issues, including synchronized blocks and methods.
• Concurrency Utilities: Use of higher-level concurrency utilities from the java.util.concurrent package.
4.4 Graphical User Interface (GUI)
• Swing: Basics of the Swing library for building graphical user interfaces, including components like JFrame, JPanel, and JButton.
• Event Handling: Handling user actions and events using event listeners and adapters.
5. Project Development
5.1
Software Development Life Cycle
• Planning and Design: Understanding the phases of software development, including requirements gathering, design, implementation, testing, and maintenance.
• Version Control: Introduction to version control systems like Git for managing code changes and collaboration.
5.2 Case Studies and Projects
• Application Development: Examples of complete projects and case studies that demonstrate the application of Java programming and data structures in real-world scenarios.
Conclusion
The 12th Edition of "Introduction to Java Programming and Data Structures" by Y. Daniel Liang provides a thorough introduction to Java programming and data structures. The book covers fundamental programming concepts, object-oriented principles, data structures, and advanced topics like exception handling, file I/O, and concurrency. It emphasizes practical applications, including GUI development and project management, making it a comprehensive resource for both beginners and intermediate programmers.