Graphql for Angular Developers: A Comprehensive Guide
In the world of web development, GraphQL has emerged as a powerful tool for building efficient and flexible APIs. Angular, a popular JavaScript framework, is widely used by developers to create dynamic and robust web applications. This comprehensive guide aims to provide Angular developers with an in-depth understanding of GraphQL and how it can be integrated into their projects. Whether you are a seasoned Angular developer or just getting started, this article will equip you with the knowledge and expertise to leverage GraphQL effectively in your Angular applications.
Graphql for Angular Developers
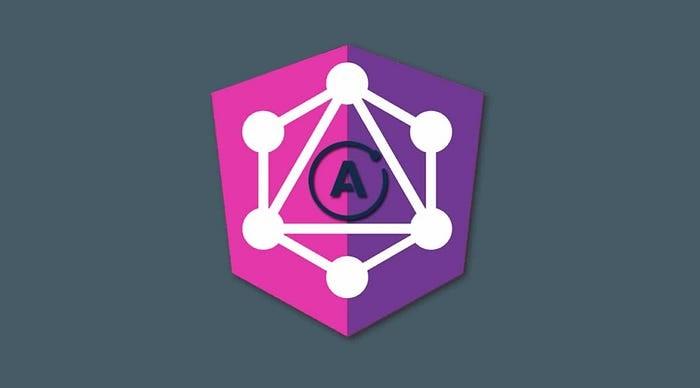
GraphQL is a query language and runtime that enables clients to request specific data from a server. Unlike traditional RESTful APIs, where clients have limited control over the data they receive, GraphQL allows clients to define the structure and shape of the data they need. This eliminates issues like over-fetching or underfetching of data, leading to more efficient and performant applications.
Why Should Angular Developers Use GraphQL?
GraphQL offers several benefits for Angular developers:
1. Efficient Data Fetching: With GraphQL API, developers can fetch multiple resources in a single request, reducing the number of network roundtrips and improving overall performance.
2. Declarative Query Language: GraphQL uses a declarative syntax that allows developers to specify exactly what data they need. This eliminates the need for multiple API endpoints and reduces the complexity of data retrieval.
3. Type Safety: GraphQL schemas provide a clear contract between the server and the client, ensuring type safety and minimizing runtime errors.
4. Rapid Iteration: GraphQL’s introspection capabilities enable developers to explore and understand the available data without relying on external documentation.
5. Real-Time Updates: GraphQL supports subscriptions, allowing clients to receive real-time updates from the server. This is particularly useful for applications that require live data updates, such as chat applications or collaborative editing tools.
Getting Started with GraphQL in Angular
To start using GraphQL in your Angular projects, you need to follow these steps:
1. Install Dependencies: Begin by installing the necessary packages. You’ll need the Apollo Client, which is a widely used GraphQL client for Angular. Install it using the following command:
bashCopy code npm install apollo-angular apollo-angular-link-http apollo-link apollolink-http graphql-tag graphql --save
1. Create GraphQL Service: Next, create a service to interact with your GraphQL server. This service will handle querying and mutating data. Use the Apollo class from apollo-angular to set up the client and connect to your GraphQL server.
2. Define GraphQL Queries: In your Angular components, define GraphQL queries to fetch data from the server. Use the apollo-angular package's Query component to execute queries and handle loading and error states.
3. Perform Mutations: To modify data on the server, use GraphQL mutations. Mutations allow you to create, update, or delete data. Use the Mutation component from apollo-angular to execute mutations
Best Practices for Using GraphQL in Angular
When using GraphQL in your Angular projects, consider the following best practices:
1. Keep Queries and Mutations Co-located: Co-locate your GraphQL queries and mutations with the corresponding Angular components. This improves maintainability and makes it easier to understand the data requirements of each component.
2. Use Fragments for Reusability: GraphQL fragments allow you to define reusable pieces of a query. Utilize fragments to avoid code duplication and improve query maintainability.
3. Leverage Apollo Caching: Apollo Client provides builtin caching capabilities that can greatly improve performance. Take advantage of caching to avoid unnecessary network requests and optimize data retrieval.
4. Implement Error Handling: GraphQL queries and mutations can fail due to various reasons, such as network errors or validation errors on the server. Implement proper error handling to provide
meaningful feedback to users and handle exceptional cases gracefully.
5. Optimize Query Efficiency: Analyze your data requirements and avoid over-fetching or under-fetching data. Use GraphQL’s advanced features like aliases, variables, and pagination to optimize query efficiency.
Leveraging GraphQL with Apollo Client
To enhance your GraphQL experience in Angular, you can leverage the power of Apollo Client. Apollo Client is a robust GraphQL client that offers advanced features such as caching, state management, and optimistic UI updates. Let’s explore how you can integrate Apollo Client into your Angular applications.
Setting Up Apollo Client in Angular
1. Install Dependencies: Start by installing the required packages for Apollo Client in your Angular project. Use the following command to install the necessary packages: bashCopy code
npm install apollo-angular apollo-client apollo-cache-inmemory graphql save
1. Configure Apollo Client: In your Angular app module, import the necessary Apollo modules and set up Apollo Client. Define the URI of your GraphQL server, create
an instance of ApolloClient, and provide it to the Angular dependency injection system.
typescriptCopy code
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
import { ApolloClient, InMemoryCache } from '@apollo/client/core';
import { ApolloModule, APOLLO_OPTIONS } from 'apollo-angular';
@NgModule({
imports: [
HttpClientModule, ApolloModule ], providers: [ {
provide: APOLLO_OPTIONS, useFactory: () => {
return {
cache: new InMemoryCache(), uri: 'https://your-graphql-server.com/graphql' }; } } ] })
export class AppModule { }
1. Executing GraphQL Queries: With Apollo Client set up, you can now execute GraphQL queries in your Angular components. Import the Apollo service from apolloangular and use the watchQuery method to execute your queries.
typescriptCopy code
import { Component } from '@angular/core';
import { Apollo } from 'apollo-angular';
import { gql } from 'apollo-angular';
@Component({
selector: 'app-example',
template: `
<div *ngIf="loading">
Loading... </div>
<div *ngIf="error">
An error occurred: {{ error.message }} </div>
<div *ngIf="data">
{{ data }} </div>
export class ExampleComponent { loading: boolean; error: any; data: any; constructor(private apollo: Apollo) { } ngOnInit() { this.apollo.watchQuery({
query: gql`
query { // Your GraphQL query here
}).valueChanges.subscribe(result => { this.loading = result.loading; this.error = result.error; this.data = result.data; });
Advantages of Apollo Client
By incorporating Apollo Client into your Angular applications, you can benefit from its powerful features:
1. Caching: Apollo Client provides an intelligent caching mechanism that automatically stores and retrieves data from the cache. This reduces the need for unnecessary network requests and improves application performance.
2. Local State Management: Apollo Client allows you to manage local application state alongside your GraphQL
data. You can define and manipulate local data using GraphQL queries and mutations, providing a unified approach to data management.
3. Optimistic UI Updates: With Apollo Client, you can implement optimistic UI updates, which means updating the UI immediately after a user action and then synchronizing the changes with the server. This provides a smooth and responsive user experience.
4. Server-Side Rendering: Apollo Client seamlessly integrates with server-side rendering (SSR) frameworks, enabling efficient rendering of GraphQL data on the server and improving initial load times.
Conclusion
By combining GraphQL and Apollo Client, Angular developers can unlock a world of possibilities for building efficient, flexible, and performant applications. This guide has equipped you with the knowledge and best practices to leverage GraphQL in your Angular projects. Additionally, integrating Apollo Client empowers you with advanced features like caching, state management, and optimistic UI updates. Embrace the power of GraphQL and Apollo Client to enhance your Angular development experience and deliver exceptional web applications.