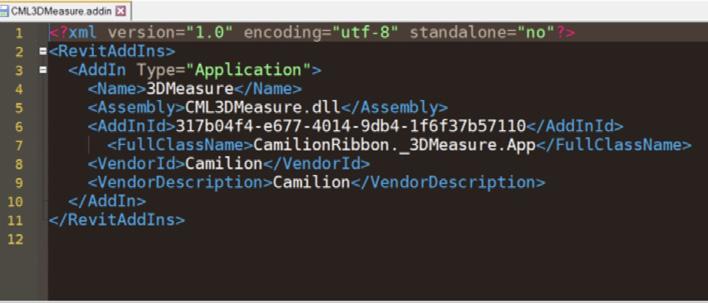
4 minute read
An introduction to creating a plugin for Revit in C#
The required software
Apart from having Revit installed, to write a Plugin in C# you’ll need to install Microsoft Visual Studio to write your C# code and compile your plugins. VS (Visual Studio) is an integrated development environment (IDE) from Microsoft. It is used to develop many kinds of computer programs, websites, web services, mobile apps, etc.
Advertisement
The required skills
To create a plugin in Revit with C# you’ll need to know:
• Revit
• C#
• The basic Revit API calls for manipulating data in Revit
• The basics of using Visual Studio to create .NET assemblies
The steps involved in creating a plugin
1. Write your code in a .NET compliant language (like C#)
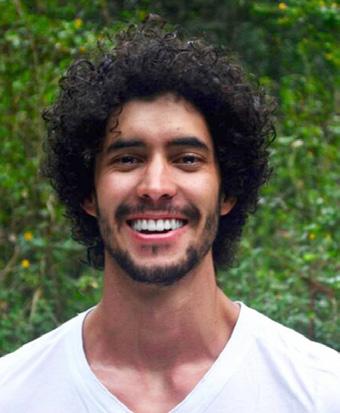
The .NET Framework is a proprietary software framework developed by Microsoft that runs primarily on Microsoft Windows.
Revit has a .NET API which means you can use any of the .NET compliant programming languages (C#, VB.NET, F#, etc.) to develop a plug-in. While each language has its own relative benefits, C# is the natural choice for most developers: it is easy-to-learn, easy-to-use and leverages the power of the underlying .NET Framework.
This step is where most of the real work is done. We can further divide this topic in the following parts:
• Create a new Project in Visual Studio: A VS project is a bunch of files structured in a specific order. This is where you’ll write your code.
• Reference the Revit API libraries: When you install Revit, you are installing the Revit API files somewhere in your computer. Referencing the Revit API means you’re telling your plugin where these files are located.
• Writing the actual code: Here you define all the functionality of your plugin, any windows, popups, warnings, the button’s appearance, tab names and so on.
• Debug your code: Debugging es the process of finding and resolving errors in the code. This is a big part of the work since there are so many variables and ways in which a person can use the plugin.
2. Compile the code into a .dll
The code you have written in the previous step is in human readable form. To make the code readable by a computer, you will need to translate it or “build” it. Once the code has successfully been built, or “compiled”, Visual Studio produces a file with a .dll extension.
This .dll file IS the actual plugin! Everything regarding the plugin is defined in this file… from how the plugin works to what icon the button (or buttons) will have. So how do we tell Revit to load this .dll to our user interface? Here’s where the Addin manifest file comes in.
3. Write the Addin manifest
An AddIn manifest is a text file located in a specific location checked by Revit when the application starts. The manifest includes information used by Revit to load and run the plug-in. An AddIn manifest has a “.addin” extension.
4. Stick the addin file in a specific location
The addin file must be placed in one of the required locations that Revit checks on startup. For debugging purposes you can use the location at: “C:\ProgramData\Autodesk\ Revit\Addins\20xx\”.
Remember that the ProgramData folder is hidden by default.
5. Fire up Revit and rejoice at the sight of your newly created plugin
If you did everything right, when you open Revit, you’ll get a warning that a new plugin has been detected and you’ll see your brandnew plugin there waiting for you to click on it!
Steppingstones to become a Revit API Ninja
Learning how to program is a very fun and rewarding activity. But if, like myself, you don’t have a formal education in computer science, it can be quite challenging to start programming with a language such as C#. For this purpose, below are the steppingstones I personally used to get there over the course of a couple of years:
• Learn Dynamo: The learning curve is short, and you get a great perspective into automating stuff in Revit.
• Learn Python: Regarded ad one of the 4 most used programming languages today, you can use it for thousands of things, from sending mails to web scraping, file management, etc.
• Use python within Dynamo: Python gives you access to the Revit API and allows you to solve problems that would be lengthy and difficult relying on Dynamo alone.
• Use Python with PyRevit: This will give you a first feeling of creating your plugins, complete with buttons and all. Plus, Ehsan, the creator of PyRevit has some great content online that can really help you understand the Revit API better.
• Learn C#: When you feel ready to jump to C#, there are innumerable free courses taught from Microsoft certified institutions (and many of them are free).
• Create your first C# plugin: The last stage of this journey. If you made it this far, you’re a Revit API ninja, congratulations!
Useful resources
These are some of the sources I personally learned from and continue to learn from every day:
• Automate the boring stuff with Python: A free e-book (also in video format) to learn the basics of the language. https:// automatetheboringstuff.com/
• PyRevit: A great (and free and opensource) plugin created and maintained by Ehsan Iran-Nejad that allows you to create your plugins in Python language. The YouTube channel has great resources to understand the Revit API. https://www.youtube.com/channel/UC0THIvKRd6n7T2a5aKYaGg
• Addin Manager (Hot Loading): While you’re debugging your code, you must open and close Revit each time you change something in the code. The Addin Manager is a plugin originally developed by Autodesk to avoid this (this is known as “Hotloading” in the developer world). This program was discontinued in 2020 but there’s an opensource alternative available online. https://github.com/ chuongmep/RevitAddInManager
• Revit’s API: Online documentation for the Revit API complete with samples and examples. https://www.revitapidocs.com/
• Autodesk’s Revit Developer Center: Official documentation for anything relating to development such as API’s, SDK’s, etc. https://www.autodesk.com/developernetwork/platform-technologies/revit
• Autodesk’s Revit API Forum: Most problems you’ll encounter during your journey have already been asked and solved by someone here. https://forums.autodesk. com/t5/revit-api-forum/bd-p/160
• The Building Coder blog: Jeremy Tammik, regarded by many as “The godfather of Revit API” has been posting solid content on the Revit API for many years. Many of your searches and frustrations will meet their end the moment you land on his blog. https:// thebuildingcoder.typepad.com/
• Archilab’s blogs: Anyone who’s used Dynamo for a while has certainly heard of Konrad Sobon through the Dynamo blog or his great Dynamo packages. His website, archi-lab.net has some great resources to get you up and running with creating a Revit plugin with buttons and all. https://archi-lab. net/building-revit-plug-ins-with-visualstudio-part-one/
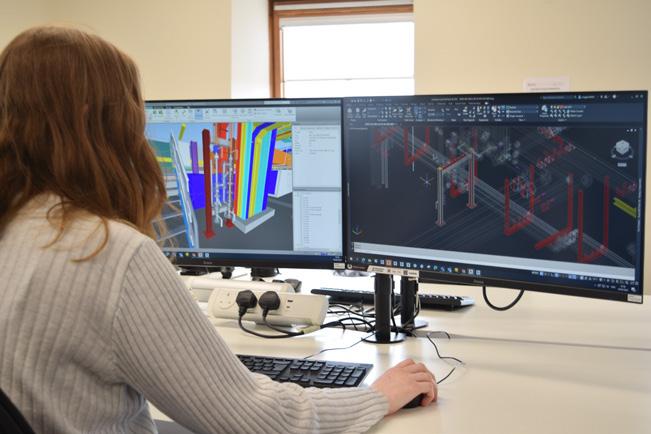
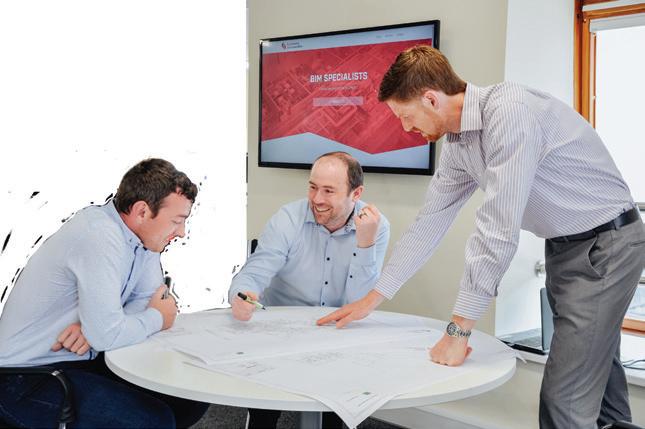
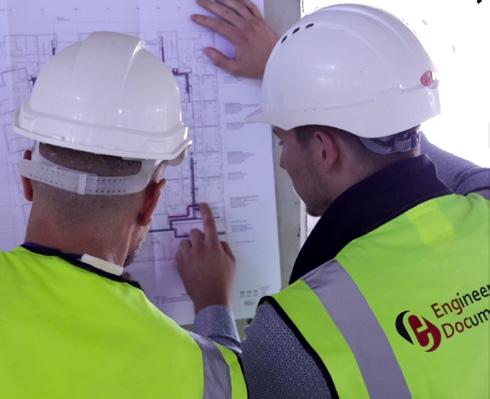
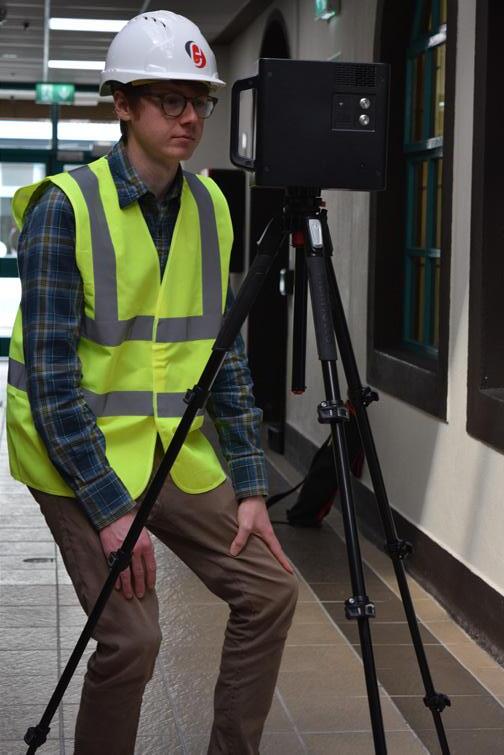